Flutter Plugin
Getting Started
Latest release:
(Changelog)
Requirements
Device Requirement


Resources
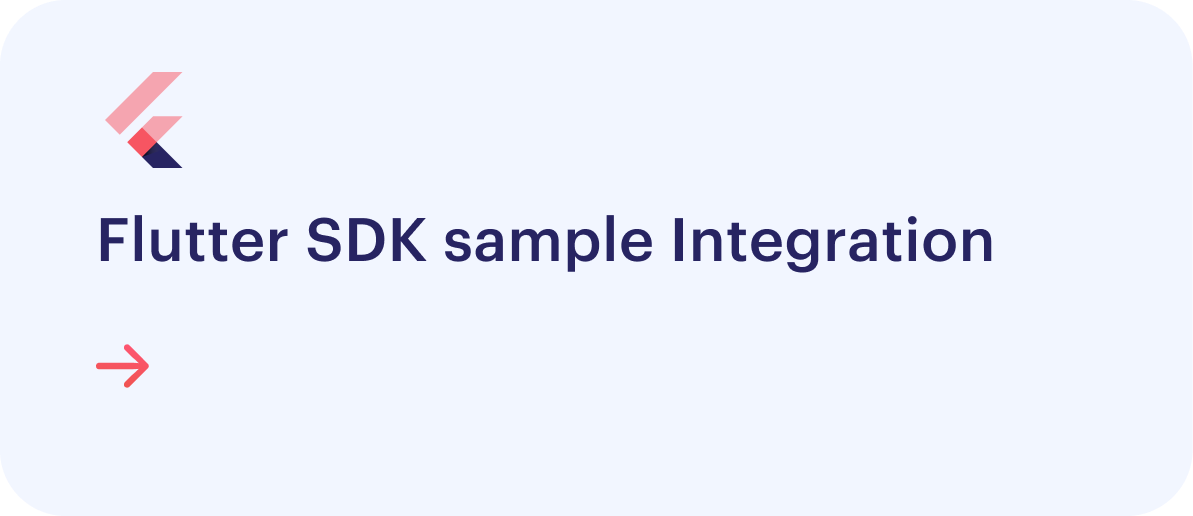
Integration
SDK Integration Guide
It’s always recommended to use the updated version
Step 1
Run this command: dart pub add shuftipro_onsite_sdk with dart or flutter pub add shuftipro_onsite_sdk with flutter


Add dependency in pubspec.yaml as
dependencies:
shuftipro_onsite_sdk: ^1.0.7
Step 2
Go to project > android > build.gradle file and add the following
allprojects {
repositories {
google()
mavenCentral()
maven { url 'https://jitpack.io' } // add this line
}
}
Step 3
Go to project > android > app > build.gradle file and add the following
android {
//Rest of your code
dataBinding {
enabled = true
}
}
Step 4
import 'package:shuftipro_onsite_sdk/shuftipro_onsite_sdk.dart' in your class to send the request to ShuftiPro's SDK.
Step 5
For iOS, navigate to the iOS folder and run the commands pod install and pod update to fetch the latest dependencies.
Step 6
For iOS, Info.plist must contain Privacy - Camera Usage Description with corresponding explanation to end-user about how the app will use these permissions
Basic Usage
Make sure you have obtained authorization credentials before proceeding. You can get client id or secret key and generate access token like this.
Authorization
The following code snippet shows how to use the access token in auth object.
Access Token
Make auth object using access token
Map<String,Object> authObject = {
"auth_type": "access_token",
"access_token": accessToken,
};
Configuration
The Shufti Pro’s mobile SDKs can be configured on the basis of parameters provided in the config object. The details of parameters can be found here
Map<String,Object> configObject = {
"base_url": "api.shuftipro.com",
"consent_age": 16,
};
Request Object
This object contains the service objects and their settings through which the merchant wants to verify end users. Complete details of service objects and their parameters can be foundhere
Map<String, Object> createdPayload = {
"country": "",
"reference": "12345678",
"language": "",
"email": "",
"verification_mode": "image_only",
"show_results": 1,
"allow_warnings": "1",
"face": {},
"document": {
"supported_types": [
"passport",
"id_card",
"driving_license",
"credit_or_debit_card",
],
/* Keep name, dob, document_number, expiry_date, issue_date empty for with-OCR request*/
"name": {
"first_name": "John",
"last_name": "Johsan",
"middle_name": "Livone",
},
"dob": "",
"document_number": "19901112",
"expiry_date": "1996-11-12",
"issue_date": "1990-11-12",
"gender": "M",
"backside_proof_required": "1",
},
//Creating Document Two Object is exactly the same as document object.
"address": {
"full_address": "ST#2, 937-B, los angeles.",
"name": {
"first_name": "Johon",
"last_name": "Johsan",
"middle_name": "Livone",
},
"supported_types": ["id_card", "utility_bill", "bank_statement", "passport", "driving_license"],
},
/* Keep name and full_address empty for with-OCR request */
"consent": {
"supported_types": ["printed", "handwritten"],
"text": "My name is John Doe and I authorise this transaction of 100",
},
};
Initialisation
Shufti Pro's mobile SDK can be initialized by using the following method and passing auth, config and request objects as the parameters.
ShuftiproSdk.sendRequest(authObject, createdPayload, configObject);
Callbacks
The SDK receives callback events as result, whether the verification journey is completed or left mid-way. The call back is received in following function
var response = await ShuftiproSdk.sendRequest(authObject, createdPayload, configObject);
The complete list of callback events can be foundhere
Obfuscation
The ShuftiPro Flutter SDK already includes the required ProGuard rules for android builds, so there's no need to add them separately.
In Android folder, Make sure you have disabled R8 full mode in the gradle.properties file in release mode.
android.enableR8.fullMode=false
Customisation
ShuftiPro supports a set of customisation options that will influence the appearance of the mobile SDK.
UI Customisation
To customize the color scheme of the entire ShuftiPro mobile SDK, including the text and background colors of widgets, update the theme settings in the Backoffice theme customization section and use the keys below for detailed modifications.
You can customize the SDK theme to match your application, choosing from Light (default), Dark, or a fully customized theme.
Light Theme
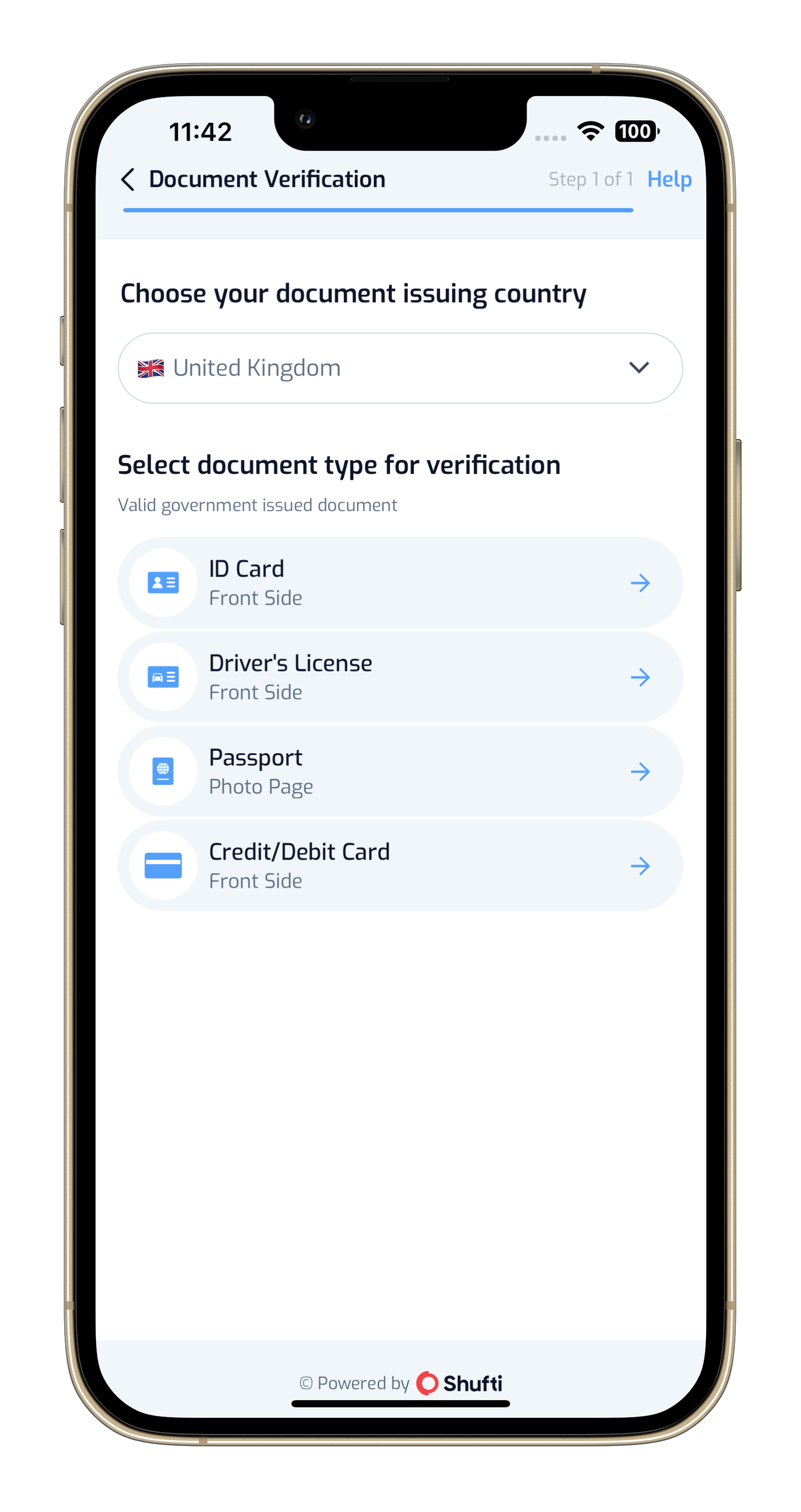
Dark Theme
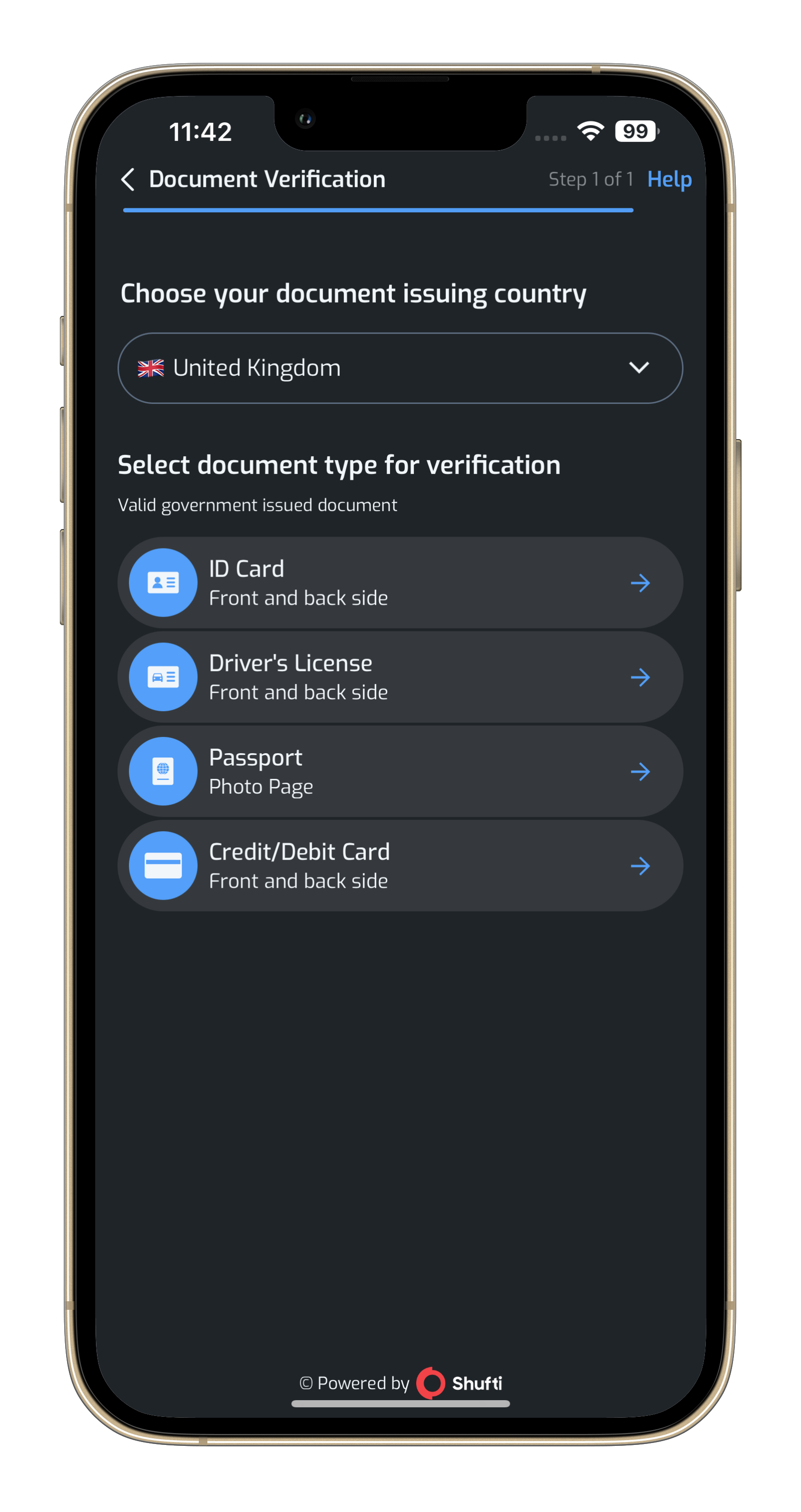
Custom Theme
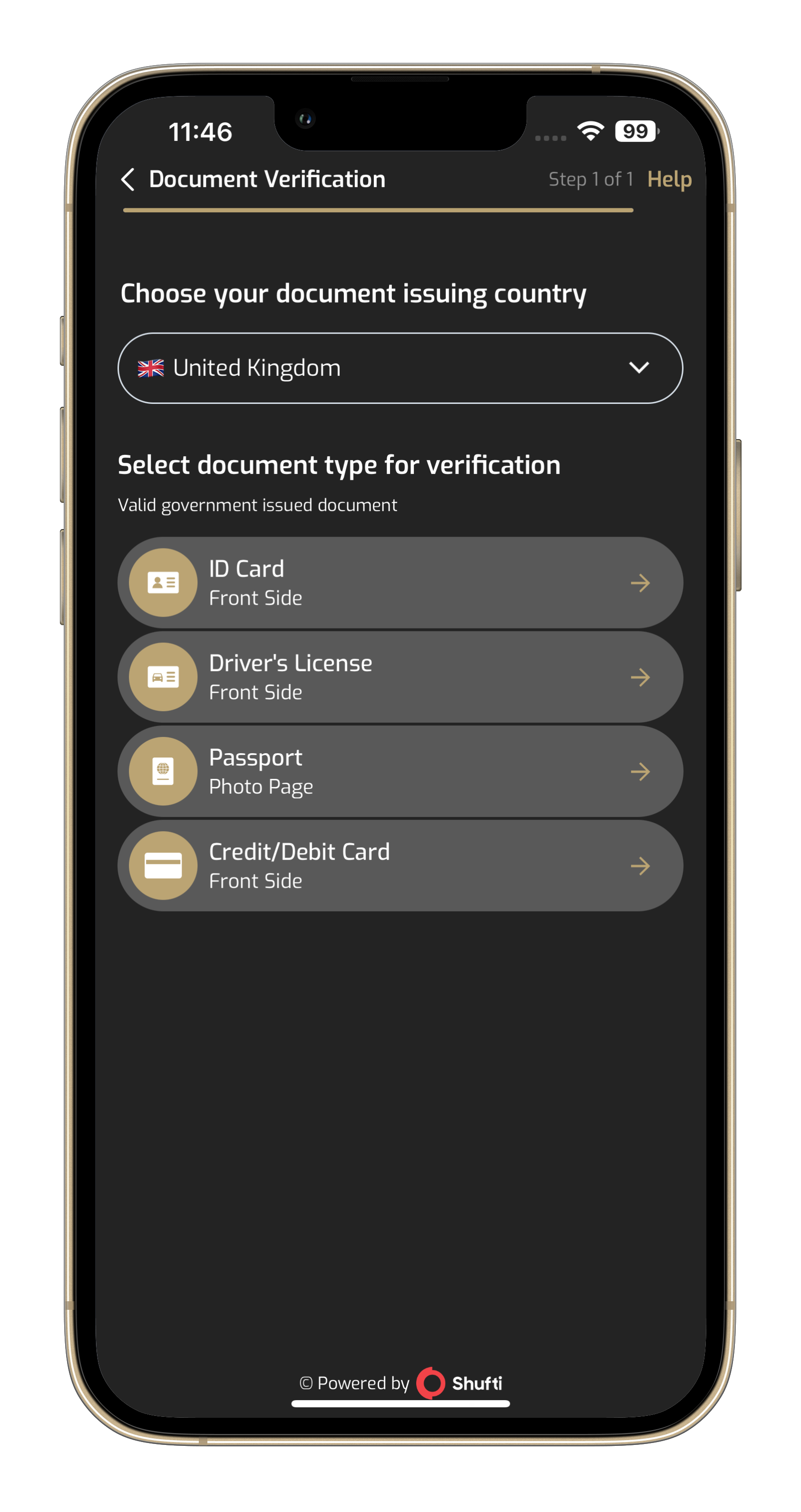
Map<String,Object> configObject = {
"icon_color": "#XXXXXX",
"theme_background_color": "#XXXXXX",
"theme_color": "#XXXXXX",
"card_background_color": "#XXXXXX",
"theme_light_color": "#XXXXXX",
"loader_color": "#XXXXXX",
"loader_text_color": "#XXXXXX",
"background_color": "#XXXXXX",
"stroke_color" : "#XXXXXX",
"dark_mode_enabled": true
};
Localization
Shufti Pro offers users the capability to customise the SDK text according to their preferred language. Merchants can modify the SDK language by specifying the language code in the country parameter within the main request object, such as "language": "en"