React Package
Getting Started
Latest release:
(Changelog)
Requirements
Device Requirement


Resources
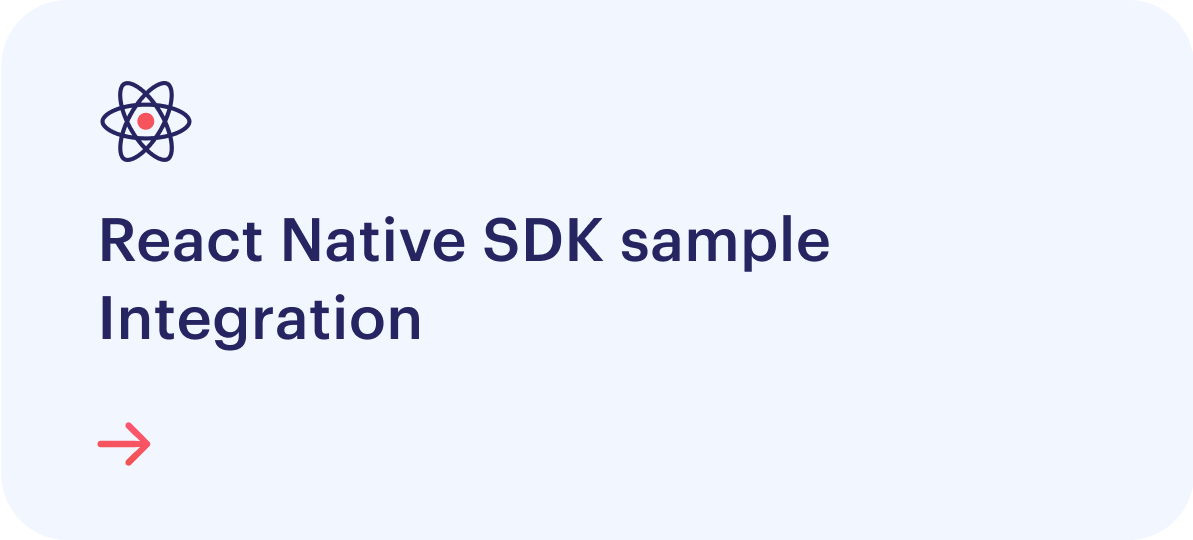
Installation
It’s always recommended to use the updated version
Step 1
Run the following command in your project terminal:
npm i shuftipro-onsite-mobilesdk
Step 2
Include the Shufti Pro module by adding the import statement at the top of your main project file.
import {NativeModules} from 'react-native';
const {ShuftiproReactNativeModule} = NativeModules;
Step 3
For Android: Go to project > android > app > build.gradle file and add the following
android {
//Rest of your code
dataBinding {
enabled = true
}
}
Step 4
For iOS, navigate to the iOS folder and run the commands pod install and pod update to fetch the latest dependencies.
Step 5
For iOS, Info.plist must contain Privacy - Camera Usage Description with corresponding explanation to end-user about how the app will use these permissions
Basic Usage
Make sure you have obtained authorization credentials before proceeding. You can get client id or secret key and generate access token like this.
Authorization
The following code snippet shows how to use the client_id and secret_key or access token in auth object.
Basic Auth
Make auth object using client id and secret key
const authObject = {
auth_type:"basic_auth",
client_id: "xxxxx-xxxxx-xxxxx",
secret_key: "xxxxx-xxxxx-xxxxx"
}
Or
Access Token
Make auth object using access token
const authObject = {
auth_type:"access_token",
access_token: "xxxxx-xxxxx-xxxxx"
}
Configuration
The Shufti Pro’s mobile SDKs can be configured on the basis of parameters provided in the config object. The details of parameters can be foundhere
const configObject = {
base_url: "api.shuftipro.com",
consent_age: 16,
};
Request Object
This object contains the service objects and their settings through which the merchant wants to verify end users. Complete details of service objects and their parameters can be foundhere
const verificationObject =
{
reference: "uniqueReference",
country: "",
language: "",
email: "",
callback_url: "",
redirect_url: "",
show_consent: 1,
show_results: 1,
allow_warnings: "1",
verification_mode:"image_only",
show_privacy_policy: 1,
face: {proof: ""},
document: {
supported_types:["passport", "id_card", "driving_license", "credit_or_debit_card"],
name: {
first_name: "",
last_name: "",
middle_name: ""
},
dob: "",
document_number: "",
expiry_date: "",
issue_date: "",
fetch_enhanced_data: "",
gender: "",
backside_proof_required: "0",
},
};
Initialisation
Shufti Pro's mobile SDK can be initialized using the given method and passing auth, config and request objects as the parameters.
ShuftiproReactNativeModule.verify(JSON.stringify(verificationObject), JSON.stringify(authObject), JSON.stringify(configObject), (res)=>{
const parsedResponse = JSON.parse(res); // Parse the JSON string into an object
const event = parsedResponse.event; // Access the value of the "event" property
console.log("Event:", event);
});
Callbacks
The SDK receives callback events as result, whether the verification journey is completed or left mid-way. The call back is received in following function
ShuftiproReactNativeModule.verify(JSON.stringify(verificationObject), JSON.stringify(authObject), JSON.stringify(configObject), (res)=>{
const parsedResponse = JSON.parse(res); // Parse the JSON string into an object
const event = parsedResponse.event; // Access the value of the "event" property
if (event === "verification.accepted") {
// Verification accepted callback
}
if (event === "verification.declined") {
// Verification declined callback
}
if (event === "verification.cancelled") {
// This callback is returned when verification is cancelled midway by the end user
}
});
The complete list of callback events can be foundhere
Obfuscation
The ShuftiPro React SDK already includes the required ProGuard rules for android builds, so there's no need to add them separately.
In Android folder, Make sure you have disabled R8 full mode in the gradle.properties file in release mode.
android.enableR8.fullMode=false
Customisation
ShuftiPro supports a set of customisation options that will influence the appearance of the mobile SDK.
UI Customisation
To customize the color scheme of the entire ShuftiPro mobile SDK, including the text and background colors of widgets, update the theme settings in the Backoffice theme customization section and use the keys below for detailed modifications.
You can customize the SDK theme to match your application, choosing from Light (default), Dark, or a fully customized theme.
Light Theme
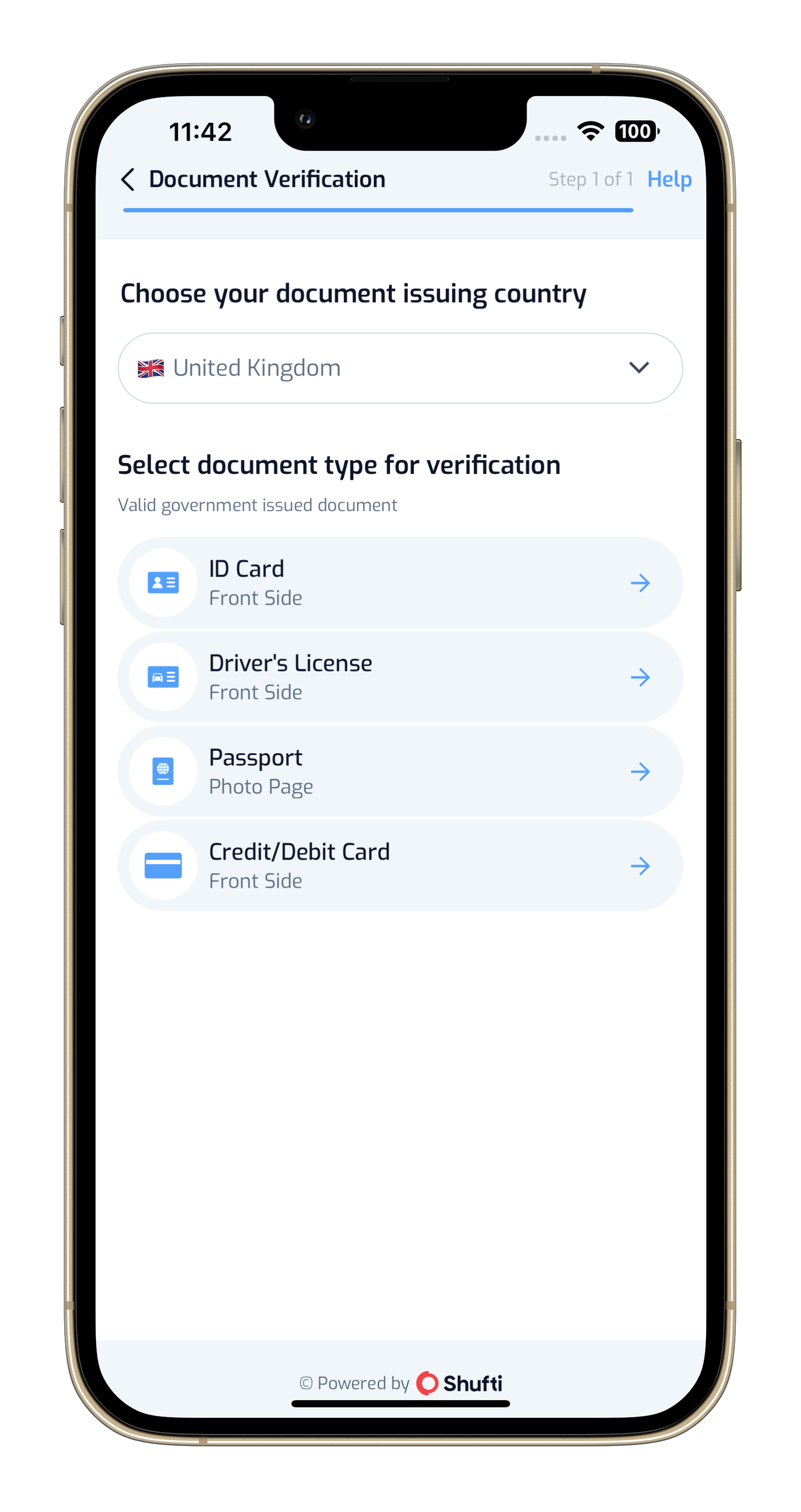
Dark Theme
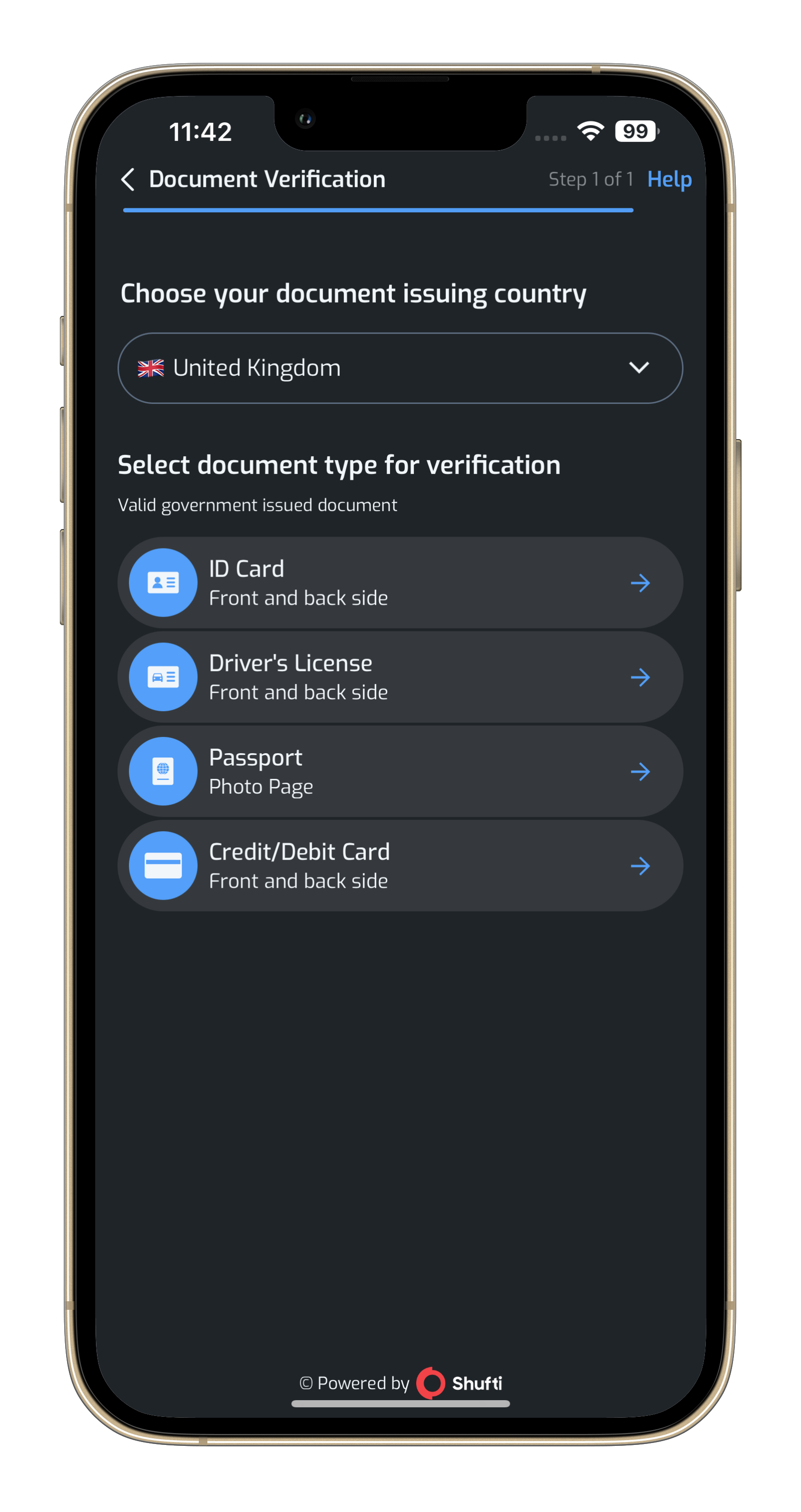
Custom Theme
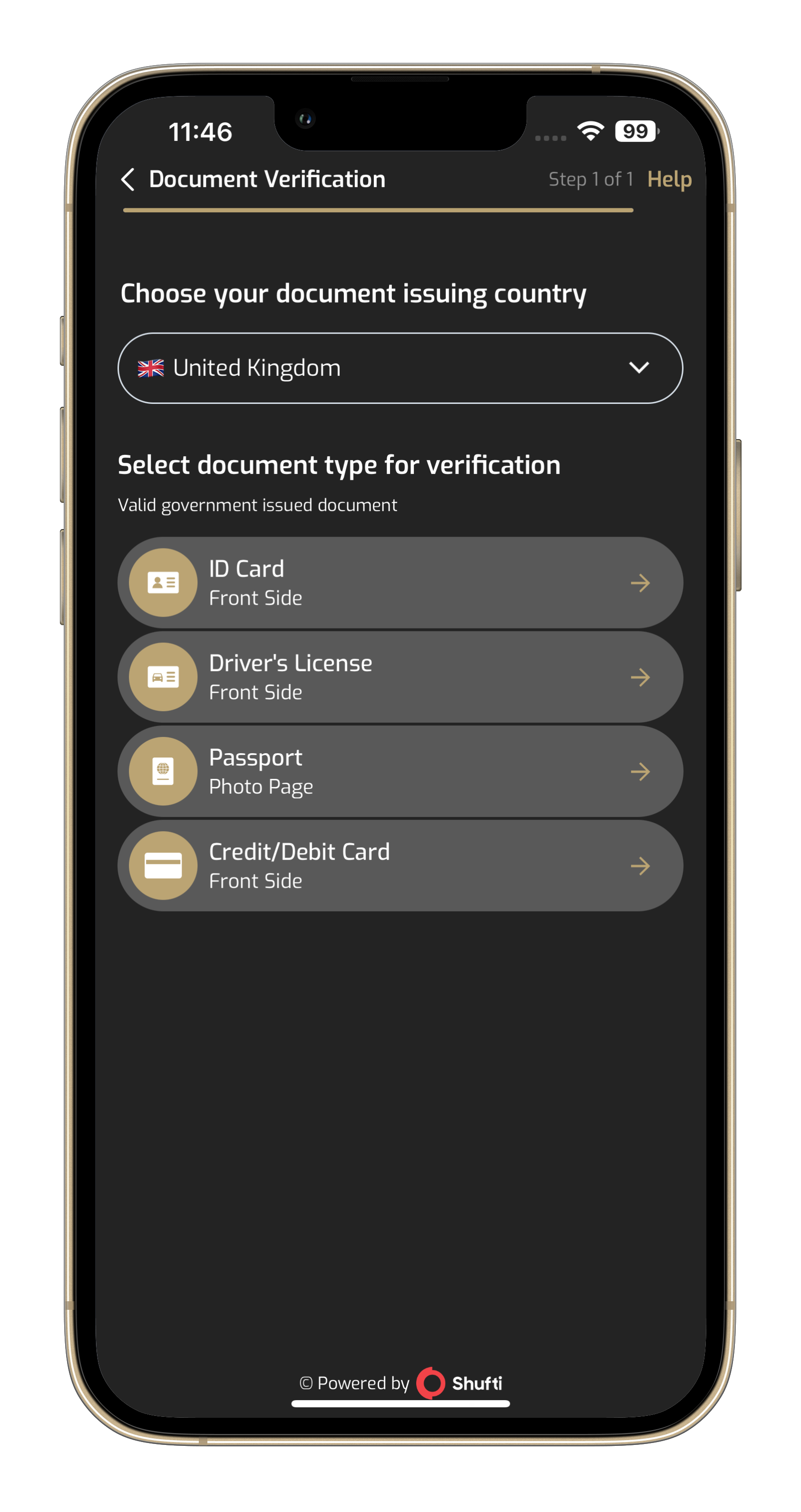
const configObject = {
icon_color: "#XXXXXX",
theme_background_color: "#XXXXXX",
theme_color: "#XXXXXX",
card_background_color: "#XXXXXX",
theme_light_color: "#XXXXXX",
loader_color: "#XXXXXX",
loader_text_color: "#XXXXXX",
background_color: "#XXXXXX",
stroke_color : "#XXXXXX",
dark_mode_enabled: true
};
Localization
Shufti Pro offers users the capability to customise the SDK text according to their preferred language. Merchants can modify the SDK language by specifying the language code in the country parameter within the main request object, such as "language": "en"
NFC verification
Near Field Communication (NFC) is a set of short-range wireless technologies. NFC allows sharing of small data payloads between an NFC tag and an NFC-supported device. NFC Chips found in modern passports, and similar identity documents contain additional encrypted information about the owner. This information is very useful in verifying the originality of a document. NFC technology helps make the verification process simple, quicker and more secure. This also provides the user with contactless and input less verification. ShuftiPro's NFC verification feature detects MRZ from the document to authenticate NFC chips and retrieve data from it, so the authenticity and originality of the provided document can be verified.
Installation
Step 1
Run the following command in your project terminal:
npm i shuftipro-onsite-mobilesdk-nfc
Step 2
Application Info.plist must contain a Privacy - Camera Usage Description and Privacy - NFC Scan Usage Description key with an explanation to the end-user about how the app uses this data.
Step 3
And Open your Info.plist file as Source Code add these lines inside the dict tag.
<key>com.apple.developer.nfc.readersession.iso7816.select-identifiers</key>
<array>
<string>00000000000000</string>
<string>A0000002471001</string>
</array>
Step 4
In your Project target, add under the Signing and Capabilities section, tap on Capabilities and add Near Field Communication Tag Reading.For more guidance watch this guided imagehere
Step 5
Please make sure to add the following post-install hook to your Podfile.
post_install do |installer|
installer.aggregate_targets.each do |target|
target.xcconfigs.each do |variant, xcconfig|
xcconfig_path = target.client_root + target.xcconfig_relative_path(variant)
IO.write(xcconfig_path, IO.read(xcconfig_path).gsub("DT_TOOLCHAIN_DIR", "TOOLCHAIN_DIR"))
end
end
installer.pods_project.targets.each do |target|
target.build_configurations.each do |config|
if config.base_configuration_reference.is_a? Xcodeproj::Project::Object::PBXFileReference
xcconfig_path = config.base_configuration_reference.real_path
IO.write(xcconfig_path, IO.read(xcconfig_path).gsub("DT_TOOLCHAIN_DIR", "TOOLCHAIN_DIR"))
end
if ['OpenSSL-Universal'].include? target.name
config.build_settings['BUILD_LIBRARY_FOR_DISTRIBUTION'] = 'YES'
config.build_settings['IPHONEOS_DEPLOYMENT_TARGET'] = '12.0'
end
end
end
end
To check NFC supported documents and countries, please clickhere
Nfc verification is allowed only on e-passports under document, document_two and address service only.