iOS SDK
Getting Started
Latest release:
(Changelog)
Requirements
Device Requirement

Resources
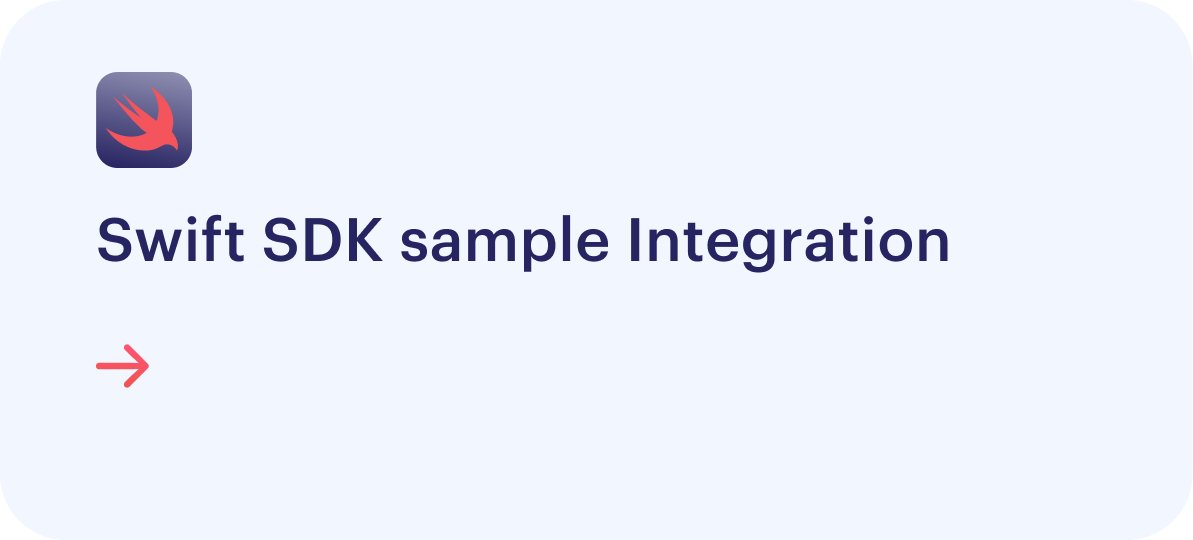
Integration
SDK Integration Guide
It’s always recommended to use the updated version and run on physical device to get real results.
- Cocoapods
Make sure your project contains pod file. If it does not, run pod init
command in root of your project directory.
Add following pod to your Podfile
pod 'ShuftiPro-Onsite'
Permissions:
Application Info.plist must contain Privacy - Camera Usage Description with corresponding explanation to end-user about how the app will use these permissions
Basic Usage
Make sure you have obtained authorization credentials before proceeding. You can get client id or secret key and generate access token like this.
Authorization
The following code snippet shows how to use the access token in auth object.
Access Token
Make auth object using access token
let authKeys = [
"auth_type" : "access_token",
"access_token" : "xxxxx-xxxxx-xxxxx"
]
Configuration
The Shufti Pro’s mobile SDKs can be configured on the basis of parameters provided in the config object. The details of parameters can be foundhere
let configs = [
"base_url": "api.shuftipro.com",
"consent_age": 16,
]
Request Object
This object contains the service objects and their settings through which the merchant wants to verify end users. Complete details of service objects and their parameters can be foundhere
Import Shufti Pro
import ShuftiPro
Request Object
let requestObject: [String: Any] = [
"reference": "Unique reference",
"country": "",
"language": "",
"email": "[email protected]",
"callback_url": "http://www.example.com",
"show_results" : "",
"allow_warnings" : "1",
"verification_mode": "image_only",
"face": ["proof": ""],
"document": [
"proof": "",
"additional_proof" :"",
"supported_types": [
"passport",
"id_card"
],
"name": [
"first_name": "",
"last_name": ""
],
"backside_proof_required": "0",
"dob": "",
"document_number": "",
"expiry_date": "",
"issue_date": ""
],
"address": [
"proof": "",
"full_address": "",
"name": [
"first_name": "",
"last_name": ""
],
"supported_types": [
"id_card",
"utility_bill",
"bank_statement"
]
],
"consent":[
"proof" : "",
"text" : "my consent note",
"supported_types" :[
"printed",
"handwritten"
]
]
]
Initialisation
Shufti Pro's mobile SDK can be initialized by using the following method and passing auth, config and request objects as the parameters.
let instance = Shufti()
instance.shuftiProVerification(requestObject: "your-request-object", authKeys: "authentication-keys-object",
parentVC: your viewController from where you want to open ShuftiPro,
configs: "configuration-object"){(result) in
print(result) // Callback response for verification verified/declined
let response = result as! NSDictionary
if response.value(forKey: "event") as? String == "verification.accepted" {
// Verified: Do something
} else {
// Declined: Do something
}
}
Callbacks
The SDK receives callback events as result, whether the verification journey is completed or left mid-way. The call back is received in following function
shufti.shuftiProVerification(requestObject: dataDictionary, authKeys: authKeys, parentVC: self, configs: configs)
{
(result) in
let reponse = result as! NSDictionary
}
The complete list of callback events can be foundhere
Callbacks Handling
All the callbacks can be handled inside the client's calling View/Screen. The response and callback handling code can be seen in the following code snippet
On SDK Completion
let reponse = result as? NSDictionary
if reponse?.value(forKey: "event") as? String == "verification.accepted" {
// Verification Accepted Callback
}
else if reponse?.value(forKey: "event") as? String == "verification.declined"{
// Verification Declined Callback
}
else if reponse?.value(forKey: "event") as? String == "request.received"{
// This event states that the verification request has been received and is under processing.
}
else if reponse?.value(forKey: "event") as? String == "request.pending"{
// This event is returned for all on-site verifications until the verification is completed or timeout.
}
On SDK Unauthentication
if reponse?.value(forKey: "event") as? String == "request.unauthorized"{
// This event occurs when the auth header is not correct and, client id/secret key may be invlaid.
}
On SDK Error
if reponse?.value(forKey: "event") as? String == "request.invalid"{
// The request invalid event occurs when the parameters specified in the request payload are not in the correct format.
}
else if reponse?.value(forKey: "event") as? String == "request.timeout"{
// This will occur if request has timed-out.
}
else if reponse?.value(forKey: "event") as? String == "permission.denied"{
// This event is returned if the user did not give camera and other permissions to sdk.
}
else if reponse?.value(forKey: "event") as? String == "verification.cancelled"{
//This event occurs when the end-user does not agree to the terms and conditions and also occur if in-between user cancel verification process.
}
Customisation
ShuftiPro supports a set of customisation options that will influence the appearance of the mobile SDK.
UI Customisation
To customize the color scheme of the entire ShuftiPro mobile SDK, including the text and background colors of widgets, update the theme settings in the Backoffice theme customization section and use the keys below for detailed modifications.
You can customize the SDK theme to match your application, choosing from Light (default), Dark, or a fully customized theme.
Light Theme
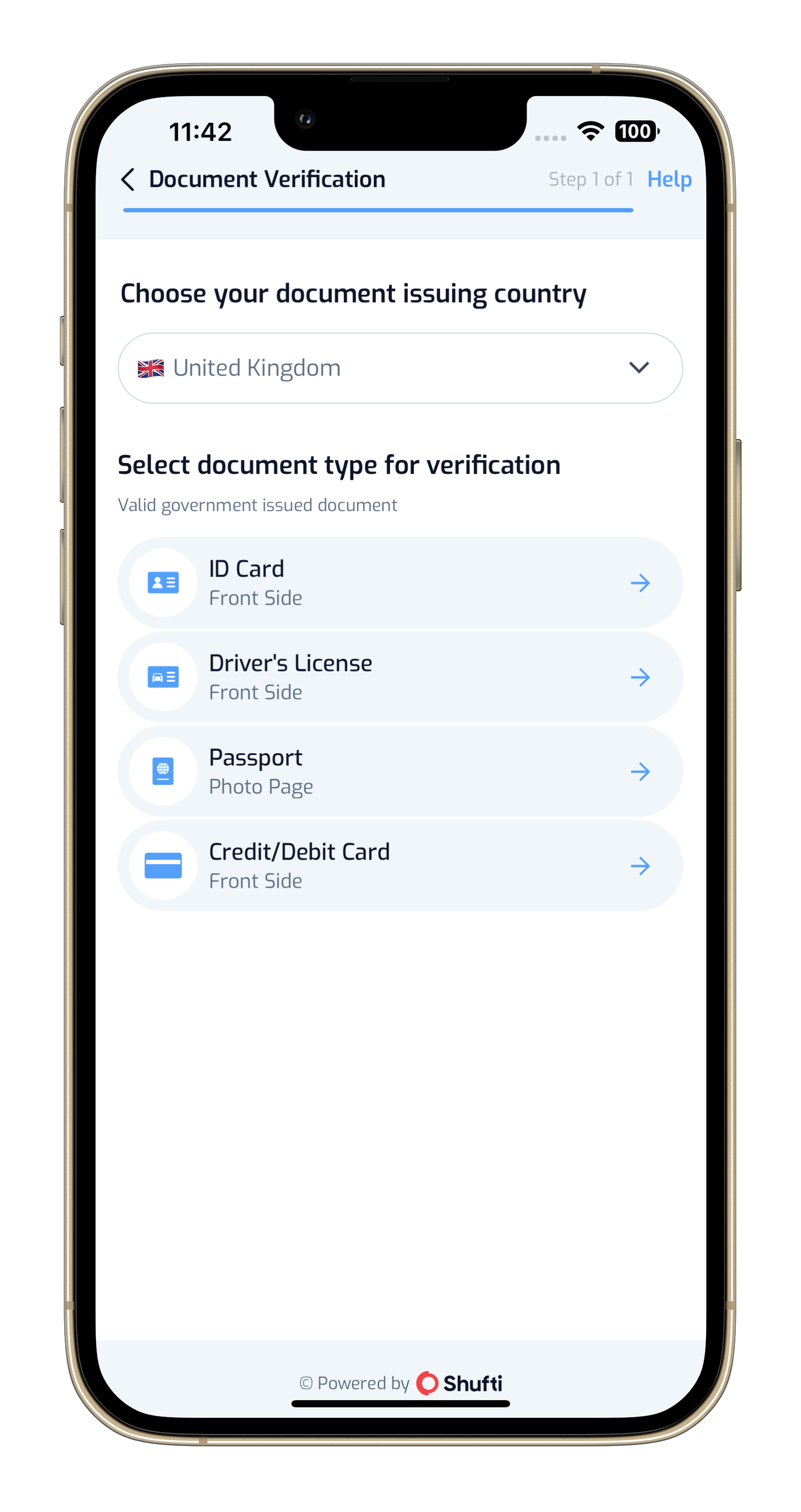
Dark Theme
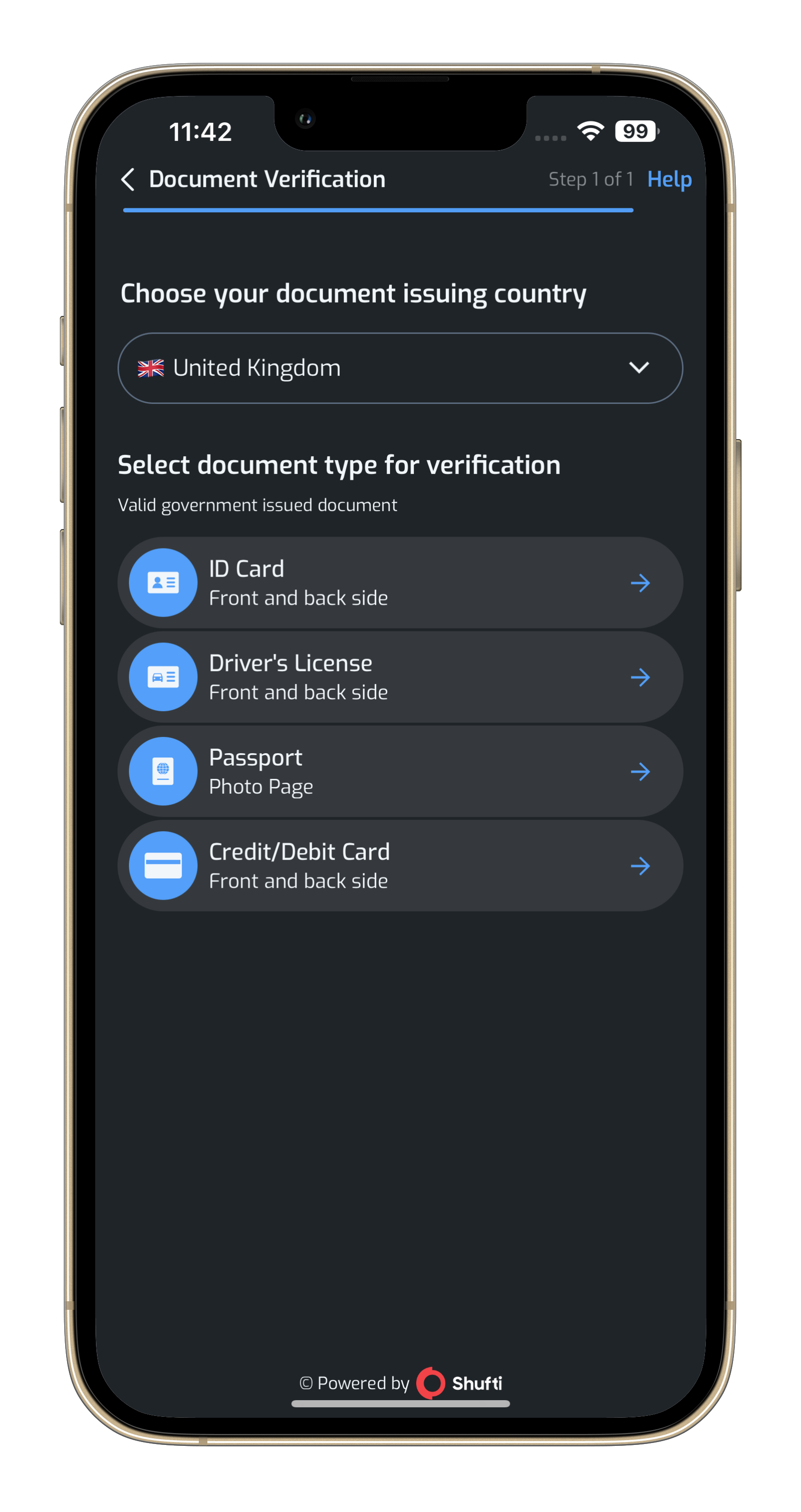
Custom Theme
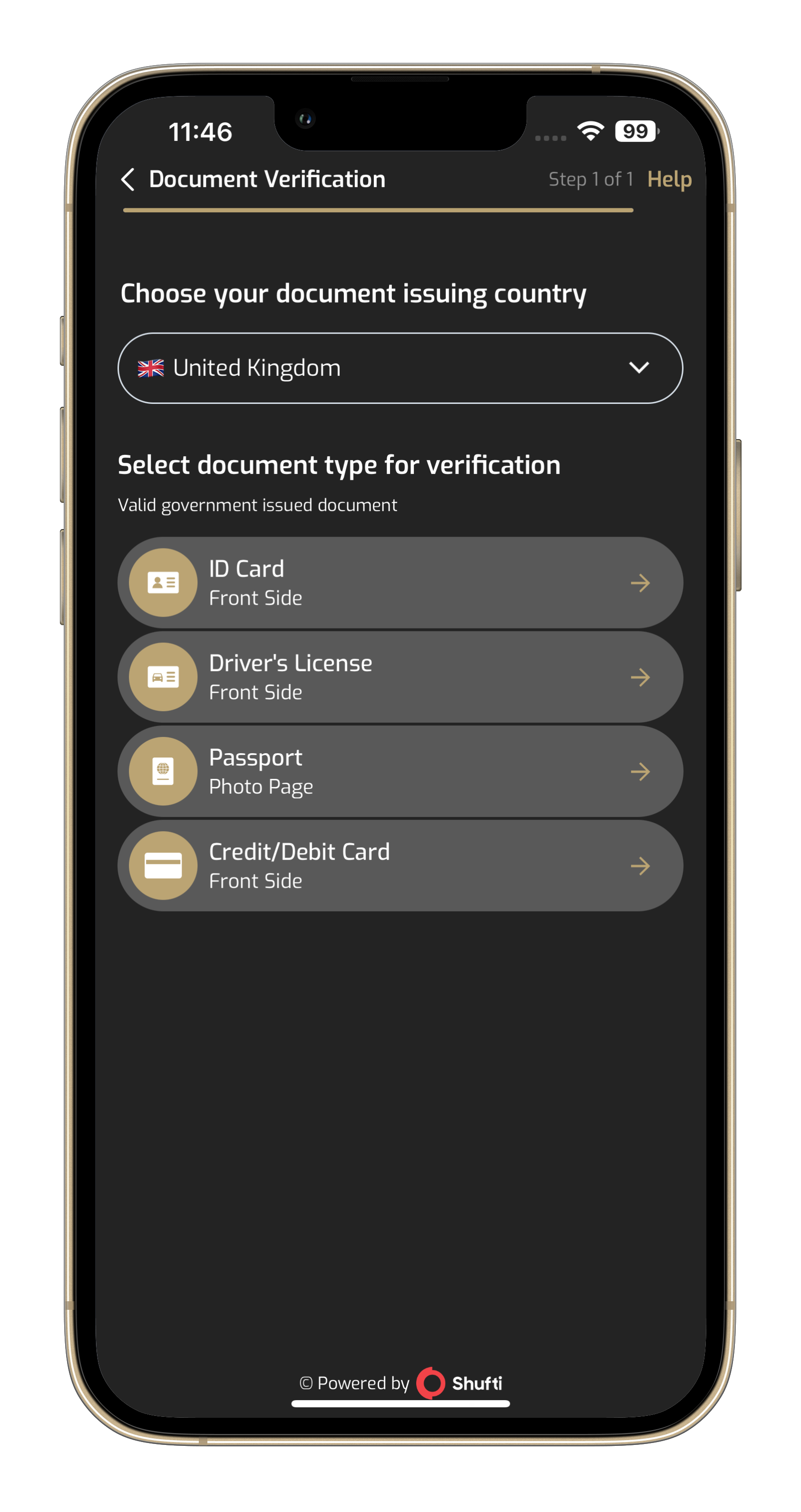
let configs = [
"icon_color": "#XXXXXX",
"theme_background_color": "#XXXXXX",
"theme_color": "#XXXXXX",
"card_background_color": "#XXXXXX",
"theme_light_color": "#XXXXXX",
"loader_color": "#XXXXXX",
"loader_text_color": "#XXXXXX",
"background_color": "#XXXXXX",
"stroke_color" : "#XXXXXX",
"dark_mode_enabled": true
]
Localization
Shufti Pro offers users the capability to customise the SDK text according to their preferred language. Merchants can modify the SDK language by specifying the language code in the country parameter within the main request object,
such as "language": "en". List of all languages are mentionhere
NFC verification
Near Field Communication (NFC) is a set of short-range wireless technologies. NFC allows sharing of small data payloads between an NFC tag and an NFC-supported device. NFC Chips found in modern passports, and similar identity documents contain additional encrypted information about the owner. This information is very useful in verifying the originality of a document. NFC technology helps make the verification process simple, quicker and more secure. This also provides the user with contactless and input less verification. ShuftiPro's NFC verification feature detects MRZ from the document to authenticate NFC chips and retrieve data from it, so the authenticity and originality of the provided document can be verified.
Installation
Step 1
Application Info.plist must contain a Privacy - Camera Usage Description and Privacy - NFC Scan Usage Description key with an explanation to the end-user about how the app uses this data.
Step 2
And Open your Info.plist file as Source Code add these lines inside the dict tag.
<key>com.apple.developer.nfc.readersession.iso7816.select-identifiers</key>
<array>
<string>00000000000000</string>
<string>A0000002471001</string>
</array>
Step 3
In your Project target, add under the Signing and Capabilities section, tap on Capabilities and add Near Field Communication Tag Reading.For more guidance watch this guided imagehere
Step 4
Add this dependency into your project's Podfile.
pod 'ShuftiProNFC'
Step 5
Please make sure to add the following post-install hook to your Podfile.
post_install do |installer|
installer.aggregate_targets.each do |target|
target.xcconfigs.each do |variant, xcconfig|
xcconfig_path = target.client_root + target.xcconfig_relative_path(variant)
IO.write(xcconfig_path, IO.read(xcconfig_path).gsub("DT_TOOLCHAIN_DIR", "TOOLCHAIN_DIR"))
end
end
installer.pods_project.targets.each do |target|
target.build_configurations.each do |config|
if config.base_configuration_reference.is_a? Xcodeproj::Project::Object::PBXFileReference
xcconfig_path = config.base_configuration_reference.real_path
IO.write(xcconfig_path, IO.read(xcconfig_path).gsub("DT_TOOLCHAIN_DIR", "TOOLCHAIN_DIR"))
end
if ['OpenSSL-Universal'].include? target.name
config.build_settings['BUILD_LIBRARY_FOR_DISTRIBUTION'] = 'YES'
config.build_settings['IPHONEOS_DEPLOYMENT_TARGET'] = '12.0'
end
end
end
end
To check NFC supported documents and countries, please clickhere
Nfc verification is allowed only on e-passports under document, document_two and address service only.
All-In-One SDK
A dynamic and efficient tool designed to transform user verification into a streamlined and effortless process. This powerful SDK enables the integration of multiple verification journeys using just a single line of code, catering to various user events and scenarios Our SDK automatically listens for the Journey you associate with a customer and initiates the verification process immediately.
Client Side
Step 1
Initialize the ShuftiPro SDK
To initialize the SDK, create a new instance of ShuftiPro and call the init method with initial data
var instance = Shufti()
instance.register(clientID: "client_id", customerID: "customer_id", configs: configs) { result in
print(result)
}
The details of parameters can be foundhere
Step 2
Destroying the SDK Instance
To clean up and destroy the SDK instance, call the destroy method.
instance.unRegister()
Server Side
Trigger verification Journey
This API triggers verification for a given customer (customer_id) with the provided verification journey (journey_id).
API Endpoint: https://api.shuftipro.com/create/customer/journey
Send the following payload to initiate the creation of a customer journey.
{
"journey_id" : "ULMTCqye1719840116",
"customer_id" : "TestingCustomer595"
"reference" : "123456789"
}
The details of parameters can be foundhere
You will receive the following response from the API call.
{
"error": false,
"message": "customer journey created successfully"
}
The details of parameters can be foundhere